Using The Entity Framework In Visual Studio With C#
Using the Entity Framework in Visual Studio facilitates the handling with database objects tremendously. It's possible to access, create, update and delete database records without using dedicated sql queries and keys. Further informations can be found on MSDN and on Wikipedia.
1. Creating a Project
For this demo purpose I create a very basic C# console application.
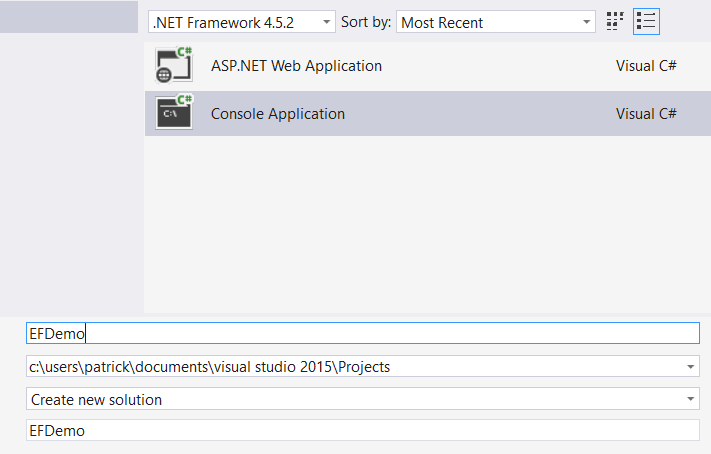
If you already have an existing application where you want to add the Entity Framework just skip the first step.
2. Adding a .edmx file
Adding the .edmx file is the key point in the whole process of using the entity framework. Within this file you can add Entities, Relationships, Properties and all the good stuff.
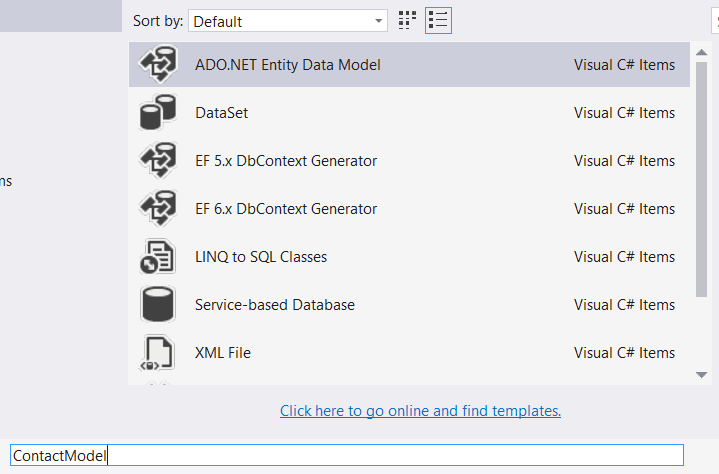
On the next page you can choose if the datamodel should be generated from an existing database or not. In our example we choose an empty model - name it ContactModel - and create our own entity in the next step.
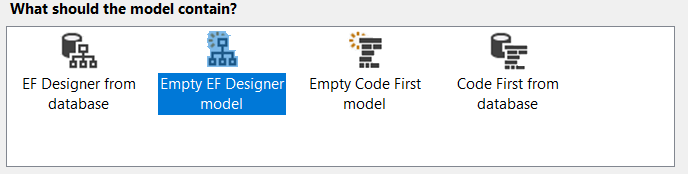
3. Creating your Entity-Relationship Model
We simply want to create a table which stores contact informations like firstname, lastname, birthdate and a nickname. First we have to add a new entity and we call it contact.
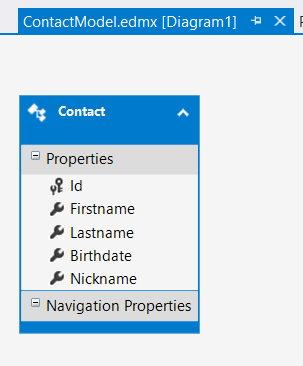
Then we can add all properties (database columns) by adding them within the shown contact entity (context menu -> add). After finishing this process Visual Studio automatically creates correspondending classes for handling those entities easily.
By right-clicking on the Entity you can also create a database script and/or set a database connection directly - which will be placed in the app.config or web.config - depending on the environment you use.
4. Work with it (incl. IntelliSense)
From now on you can use the ContactModelContainer for accessing a real database, to add (insert) new database records, to read them and so on. In my example I am adding 100 contacts with different names (by adding the index of the for loop) and nearly the same birthdate - the actual time minus 28 years.
static void Main(string[] args)
{
ContactModelContainer cmc = new ContactModelContainer();
for (int i = 0; i < 100; i++)
{
Contact contact = new Contact
{
Firstname = $"Firstname {i}",
Lastname = $"Lastname {i}",
Birthdate = DateTime.Now.AddYears(-28),
Nickname = $"Nickname {i}",
};
cmc.Contacts.Add(contact);
}
cmc.SaveChanges();
}
After running this program my database table of contacts will look like this (only the first 12 rows are shown):
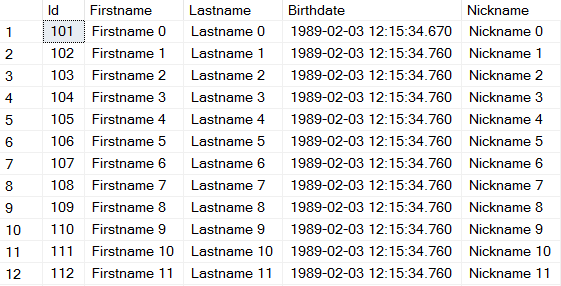
And that is basically everything you need to do when you want to work with the Entity Framework - which i highly recommend compared to working with plain SQL scripts or something like that.